To build production-ready applications with React, you need to install Node.js and npm. These tools allow you to manage dependencies and run React projects smoothly.
Quick Overview of React #
You can try React directly in an HTML file to get a feel for it. This is useful for testing or learning but is not recommended for production applications. For professional projects, you’ll need to set up a React environment.
Using React Directly in HTML #
The fastest way to start learning React is by including it directly in an HTML file using CDNs. Below is an example of how to write React code directly in HTML:
Example: React in HTML #
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>React in HTML | Coaching Wallah</title>
<!-- React and ReactDOM Scripts -->
<script src="https://unpkg.com/react@18/umd/react.development.js" crossorigin></script>
<script src="https://unpkg.com/react-dom@18/umd/react-dom.development.js" crossorigin></script>
<script src="https://unpkg.com/@babel/standalone/babel.min.js"></script>
</head>
<body>
<div id="root"></div>
<script type="text/babel">
function Welcome() {
return <h1>Hello from Coaching Wallah!</h1>;
}
const rootElement = document.getElementById('root');
const root = ReactDOM.createRoot(rootElement);
root.render(<Welcome />);
</script>
</body>
</html>
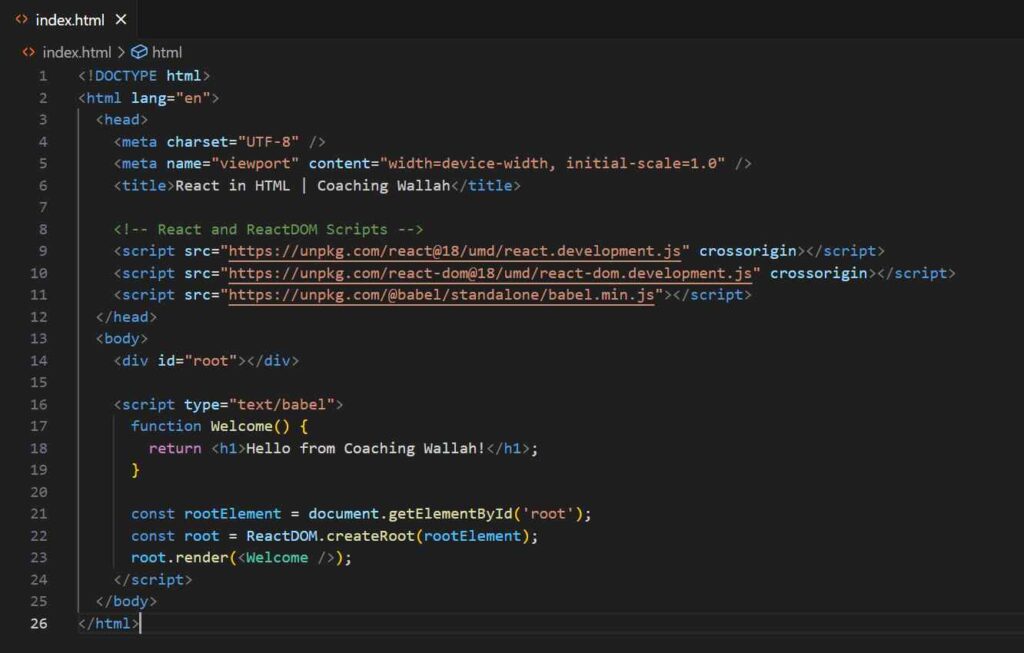
Explanation: #
- React and ReactDOM Scripts: These libraries enable React to render content inside the browser.
- Babel Script: Allows us to use JSX (JavaScript XML) syntax in modern browsers.
- React Component: The
Welcome
function returns a simple<h1>
element. - Rendering the Component:
ReactDOM.createRoot()
renders the component inside the<div id="root">
.
This approach works for practice or demos, but for professional use, you should set up a full React environment.
Setting Up a React Environment #
Prerequisites: #
- Node.js and npm: Install them from Node.js official website.
Step 1: Create a New React App #
Use the following command to create a new React project:
npx create-react-app my-react-app
Note: If you have previously installed create-react-app
globally, uninstall it first to avoid issues:
npm uninstall -g create-react-app
Step 2: Navigate to the Project Directory #
Move into the newly created project folder:
cd my-react-app
Step 3: Start the Development Server #
Run the following command to start the React app:
npm start
This will launch your app in the browser at http://localhost:3000
. If it doesn’t open automatically, type the address manually in your browser.
Modifying the React Application #
The default React app includes several files inside the src
folder. The main file is App.js. Let’s look at how we can modify the default content.
Default App.js
File: #
import logo from './logo.svg';
import './App.css';
function App() {
return (
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<p>Edit <code>src/App.js</code> and save to reload.</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React
</a>
</header>
</div>
);
}
export default App;
Modify the Content: #
To change the content, replace everything inside <div className="App">
with a custom <h1>
element:
function App() {
return (
<div className="App">
<h1>Hello World from Coaching Wallah!</h1>
</div>
);
}
export default App;
Note: You can remove the unnecessary imports like logo.svg
and App.css
if they are not required.
Simplify Your React Application #
You can also simplify the project by editing index.js to directly render your first element. Here’s an example:
index.js
Example: #
import React from 'react';
import ReactDOM from 'react-dom/client';
const myElement = <h1>Hello React!</h1>;
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(myElement);
Explanation: #
- ReactDOM.createRoot(): This method initializes the React app.
- myElement: The JSX element that will be rendered inside the
root
div.
What’s Next? #
Now that your React environment is up and running, you’re ready to dive deeper into React components, state management, and other key concepts. Keep exploring and build amazing projects with React on Coaching Wallah!
Want to learn React with an instructor, either offline or online? Find experienced tutors near you and join Coaching Wallah—completely free for students!
Leave your comment