What is React? #
React is a JavaScript library developed by Facebook for building interactive and reusable user interface components. It’s often mistaken for a framework due to its powerful capabilities, but it focuses primarily on the view layer in web applications.
React allows developers to build large web applications that can update data without reloading the entire page, making it perfect for dynamic user interfaces.
How Does React Work? #
React relies on something called the Virtual DOM to make updates fast and efficient. Here’s how it works:
- Instead of directly updating the browser’s DOM, React creates a virtual DOM – a lightweight copy of the actual DOM.
- It performs all necessary changes in the virtual DOM first.
- Once the updates are finalized, React determines which parts of the actual DOM need to be changed and only updates those parts.
This approach ensures that the application stays fast and smooth, even as the user interacts with it.
Example: React Component #
Here is a simple example of a React component that renders a greeting message on the screen:
// Import React library
import React from 'react';
import ReactDOM from 'react-dom';
// Create a simple component
function Greeting() {
return <h1>Hello, welcome to Coaching Wallah!</h1>;
}
// Render the component to the browser DOM
ReactDOM.render(<Greeting />, document.getElementById('root'));
Explanation: #
- Greeting Component: A functional component that returns a heading.
- ReactDOM.render(): This function renders the
Greeting
component inside the HTML element with the IDroot
.
History of React.js #
- 2011: Initially developed for Facebook’s Newsfeed feature.
- July 2013: React was released to the public (v0.3.0).
- April 2022: The latest stable version, v18.0.0, was released.
- create-react-app: This tool simplifies setting up a React project by including necessary build tools like Webpack, Babel, and ESLint. The latest version of create-react-app is v5.0.1 (April 2022).
Setting up React with create-react-app
#
You can quickly create a new React project using the create-react-app
tool. Follow these steps:
Step 1: Install Node.js #
Make sure you have Node.js installed. You can download it from Node.js official website.
Install Create-React-App Tool #
The next step is to install a tool called create-react-app using NPM. This tool is used to create react applications easily from our system. You can install this at the system level or temporarily at a folder level. We will install it globally by using the following command.
npm install -g create-react-app
Step 2: Create a New React Project #
Open your terminal and run the following command:
npx create-react-app my-app
This command creates a new React project named my-app
.
Step 3: Navigate to Your Project Folder #
cd my-app
Step 4: Start the Development Server #
npm start
This will start your React application at http://localhost:3000/
. You should see the default React welcome screen.
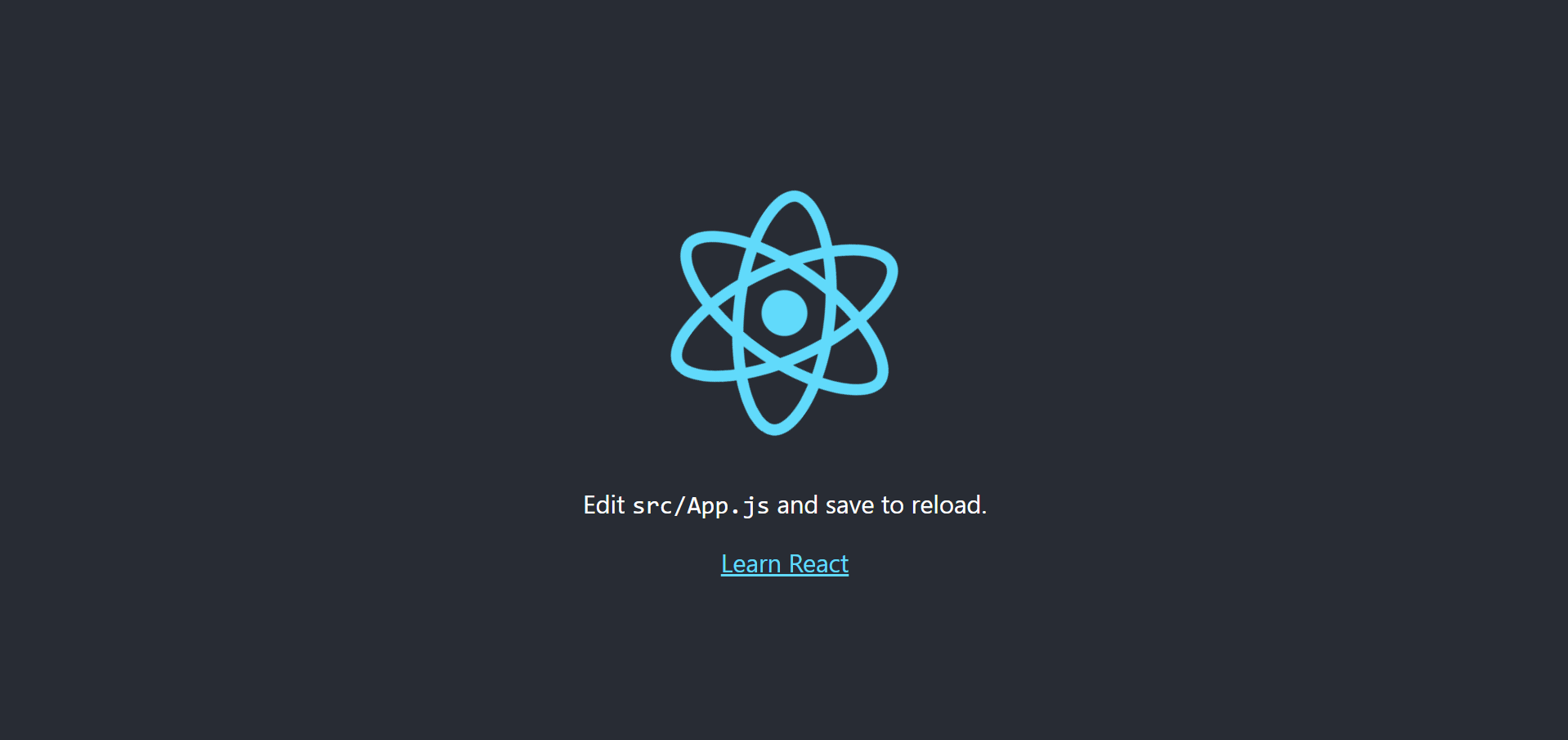
React is a versatile and powerful JavaScript library that simplifies the process of creating interactive UIs. Its use of the virtual DOM makes updates efficient, and tools like create-react-app make it easy to get started.
Want to learn React with an instructor, either offline or online? Find experienced tutors near you and join Coaching Wallah—completely free for students!
Leave your comment